Understanding the differences between structured, procedural, and object-oriented programming (OOP) is crucial for any aspiring programmer. These programming paradigms offer distinct approaches to organizing and designing code, each with its own strengths and weaknesses. This guide will delve into the key characteristics of each paradigm, their benefits, and common use cases to help you choose the right approach for your next project.
Structured vs Procedural vs Oriented Programming
1. Structured Programming: The Foundation of Orderly Code
Structured programming is a paradigm emphasizing code organization and readability. It advocates breaking down complex problems into smaller, more manageable modules or functions. Key features of structured programming include:
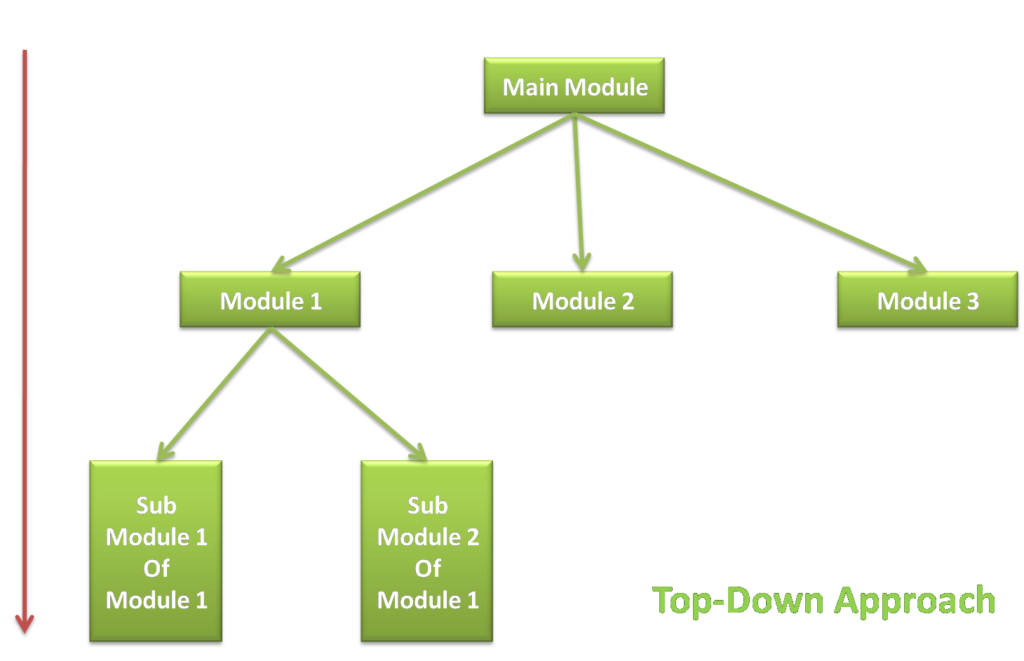
- Top-Down Design: The problem is decomposed into a hierarchy of sub-problems, making it easier to understand and manage complexity.
- Modularity: Code is organized into reusable functions, promoting code reusability and maintainability.
- Control Structures: Conditional statements (if/else) and loops (for, while) control the flow of execution, making code more predictable.
- Avoidance of GOTO Statements: Instead of using unstructured jumps, structured programming relies on clear control flow mechanisms.
2. Procedural Programming: Step-by-Step Problem Solving
Procedural programming is an extension of structured programming, focusing on procedures (functions) as the primary building blocks of code. Here’s how it differs:
- Sequence of Actions: Procedural programming emphasizes a step-by-step approach to solving problems.
- Data and Functions: Data and functions are treated as separate entities.
- Global Data: Data is often shared globally across functions, which can lead to unintended side effects.
- Examples: C and Pascal are classic examples of procedural languages.
3. Object-Oriented Programming (OOP): Modeling the Real World
Object-oriented programming takes a different approach, modeling software as a collection of interacting objects. Key principles of OOP include:
- Encapsulation: Bundling data and functions together into objects, hiding internal details and providing controlled access.
- Inheritance: Creating new classes (blueprints for objects) based on existing ones, inheriting properties and behaviors.
- Polymorphism: The ability for objects of different classes to be treated as if they were of the same type.
- Examples: Java, C++, Python, and C# are popular OOP languages.
Choosing the Right Paradigm: A Matter of Purpose
- Structured Programming: Suitable for small to medium-sized projects where clear structure and readability are paramount.
- Procedural Programming: Well-suited for tasks that can be broken down into a clear sequence of steps.
- Object-Oriented Programming: Ideal for large, complex projects where code reusability, modularity, and maintainability are critical.
Frequently Asked Questions (FAQ):
Q: Which paradigm is best for beginners?
A: Structured programming is often recommended for beginners due to its simplicity and emphasis on organized code.
Q: Can I mix different paradigms in my code?
A: Yes, many languages allow you to blend elements of different paradigms. For example, you can use object-oriented design within a procedural language like C.
Q: Are there any other programming paradigms besides these three?
A: Yes, there are several other paradigms, such as functional programming, logic programming, and aspect-oriented programming.
Q: Which paradigm is used in web development?
A: Web development often involves a combination of OOP (for backend logic) and procedural programming (for frontend scripting).