Real World Applications of the Stack are numerous and integral to both computing and everyday problem-solving. A stack is a linear data structure that follows the Last In, First Out (LIFO) principle. This unique behavior is leveraged in many areas, from software development to real-world systems, making stacks a cornerstone of data management and operations.
Table of Contents
What is a Stack?
A stack is a collection of elements where operations like addition (push) and removal (pop) are performed at one end, known as the top of the stack. This structure ensures that the most recently added item is the first to be removed.
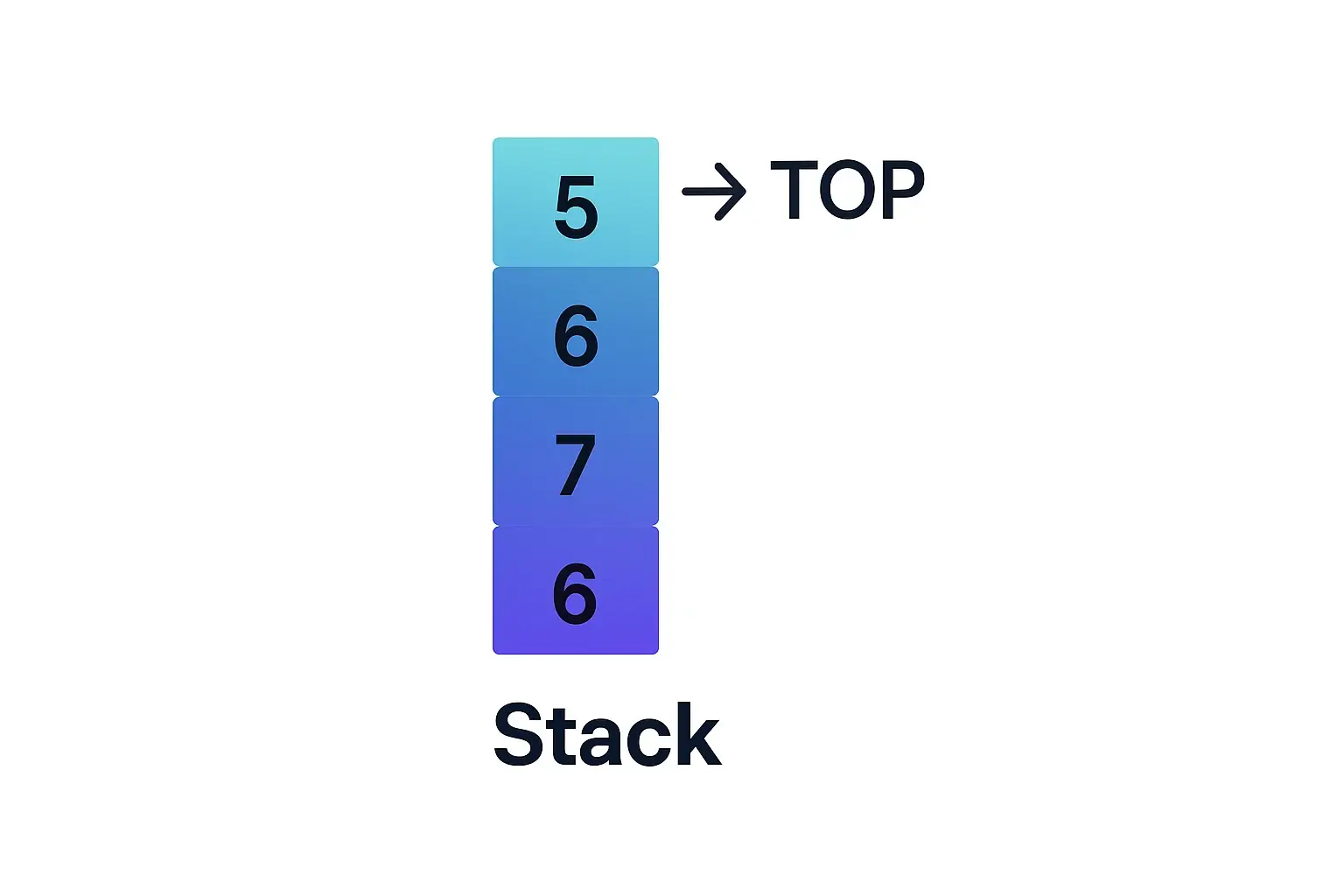
Key Features of a Stack
- LIFO Structure: Last In, First Out operation order.
- Push Operation: Adding an item to the stack.
- Pop Operation: Removing the top item from the stack.
- Peek Operation: Viewing the top item without removing it.
Real World Applications of the Stack
1. Expression Evaluation and Conversion
Stacks play a pivotal role in evaluating mathematical expressions and converting them between different notations.
Infix to Postfix Conversion
Infix expressions like A + B
are not directly computable by machines. Stacks simplify the conversion to postfix (e.g., AB+
), enabling easier computation.
Expression Evaluation
Postfix and prefix expressions are evaluated using stacks, ensuring efficient and error-free calculations.
2. Backtracking Algorithms
Backtracking relies heavily on the stack data structure to manage decision points during exploration.
Examples of Backtracking
- Maze Solving: Stacks keep track of visited paths and help retrace steps when a dead end is encountered.
- Game State Exploration: In games like chess or Sudoku, stacks help analyze moves and undo actions as needed.
3. Function Call Management
In programming, the stack is crucial for managing function calls and returns.
Call Stack
- When a function is called, its local variables and execution state are stored on the stack.
- Once the function completes, the stack removes its data and resumes the previous function.
This process enables recursive functions and nested calls to work seamlessly.
4. Browser Navigation
Web browsers use stacks to manage user navigation history.
How it Works
- Backward Navigation: Pages visited are pushed onto the stack. Moving back pops the current page and shows the previous one.
- Forward Navigation: A separate stack maintains forward pages, allowing users to revisit them easily.
5. Undo Mechanisms
Applications like text editors, image editors, and even IDEs use stacks to implement undo and redo functionality.
Example: Text Editor
- Undo: Each edit is pushed onto the undo stack. Undoing pops the last change.
- Redo: Undone changes are pushed onto a redo stack for reapplication.
6. Parsing in Compilers
Stacks are instrumental in parsing programming languages and validating syntax.
Parenthesis Matching
Stacks ensure that parentheses, brackets, and braces in code are correctly matched and nested.
Syntax Trees
Compilers use stacks to build abstract syntax trees, simplifying code compilation and execution.
7. Memory Management
Stacks are vital for efficient memory allocation and deallocation during program execution.
Stack Frames
Each function call creates a new stack frame containing its local variables and parameters. This frame is automatically discarded when the function completes, ensuring efficient memory use.
Advantages of Using Stacks
- Efficient Operations: Push and pop are constant-time operations.
- Predictable Behavior: The LIFO order simplifies implementation and debugging.
- Versatility: Applicable in diverse scenarios, from algorithms to real-world systems.
Disadvantages of Stacks
- Limited Size: Fixed-size stacks can lead to overflow if not managed properly.
- Lack of Random Access: Elements can only be accessed sequentially, limiting flexibility.
FAQs on Real World Applications of the Stack
1. What are the most common uses of stacks in everyday applications?
Stacks are commonly used in browser navigation, undo mechanisms, and function call management.
2. How does a stack differ from a queue?
A stack operates on a LIFO basis, while a queue follows First In, First Out (FIFO) principles.
3. Why are stacks important in recursion?
Recursion relies on the stack to store intermediate states and manage return values effectively.
4. Can stacks be implemented using arrays?
Yes, stacks can be implemented using arrays or linked lists, depending on the required flexibility and memory constraints.
5. What is a real-life analogy for a stack?
A stack is similar to a stack of plates in a cafeteria, where you can only add or remove plates from the top.
The versatility of stacks makes them indispensable in both computing and real-world scenarios. By understanding real world applications of the stack, you can appreciate their significance and effectively apply them to solve complex problems.