Decision control instructions are the backbone of any programming language, enabling your code to make intelligent choices and respond to varying conditions. In C, you wield three potent decision-making tools: the if
statement, the if-else
statement, and the switch
statement. This comprehensive guide will empower you to harness these tools, crafting C programs that adapt and react to diverse scenarios with precision.
Why Decision Control Instructions Are Essential
In real life, we make countless decisions every day, altering our actions based on circumstances. Similarly, your C programs must also possess the ability to evaluate conditions and choose different paths of execution. Decision control instructions empower you to create dynamic, responsive software that adapts its behavior based on user input, data values, or any other factor you define.
1. The if
Statement: Basic Conditional Execution
The if
statement is the simplest decision control instruction in C. It evaluates a condition and, if the condition is true, executes a block of code.
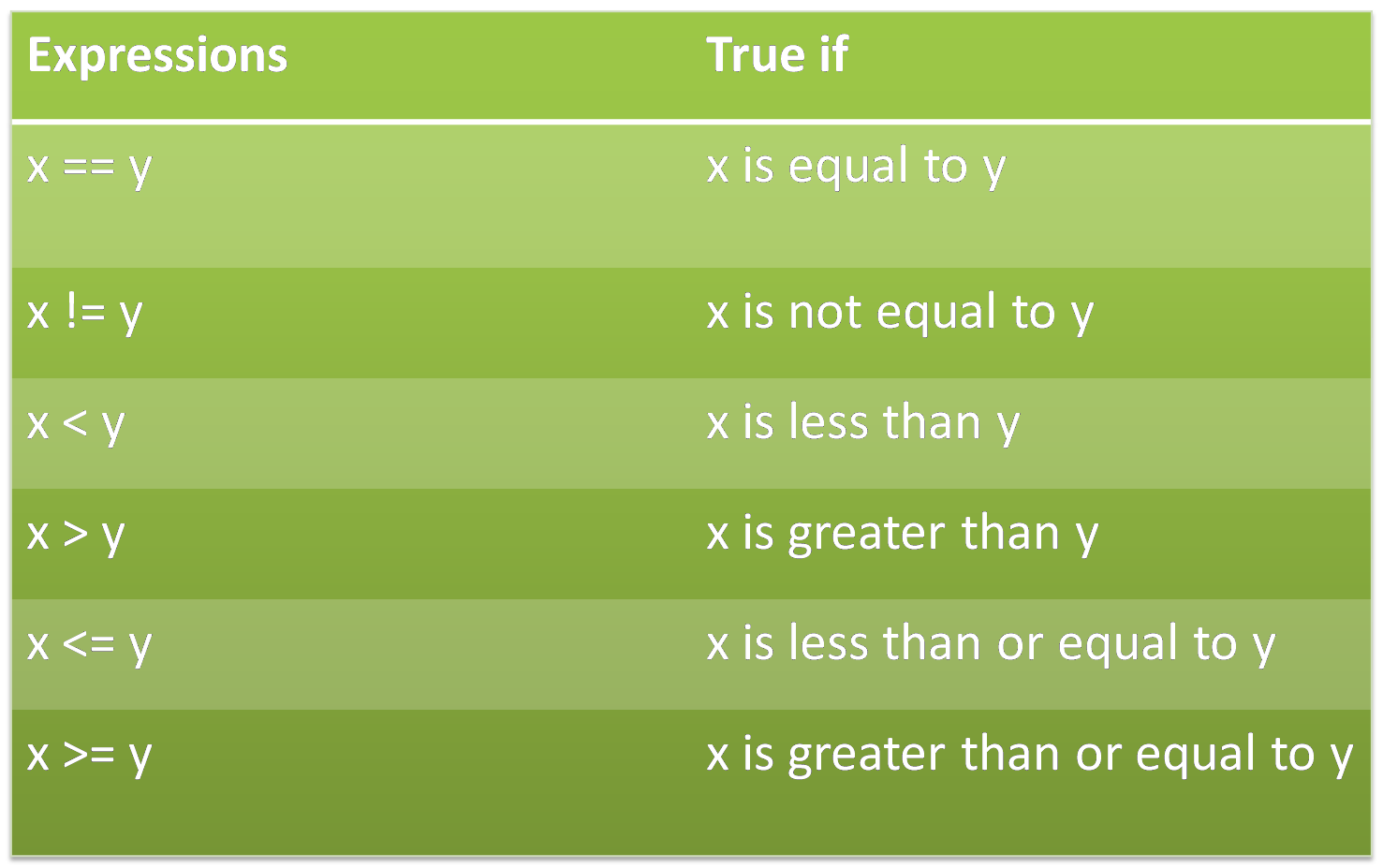
Syntax:
if (condition) {
// Code to execute if the condition is true
}
Example:
int age = 25;
if (age >= 18) {
printf("You are eligible to vote.\n");
}
2. The if-else
Statement: Handling Multiple Possibilities
The if-else
statement extends the if
statement by providing an alternative code path if the condition is false.
Syntax:
if (condition) {
// Code to execute if the condition is true
} else {
// Code to execute if the condition is false
}
Example:
int number = -5;
if (number > 0) {
printf("Positive number\n");
} else {
printf("Negative number or zero\n");
}
3. The switch
Statement: Streamlining Multiple Choices
The switch
statement is ideal for scenarios where you have multiple discrete cases to handle. It compares the value of an expression to multiple constant values, executing the code block associated with the matching case.
Syntax:
switch (expression) {
case value1:
// Code to execute if expression matches value1
break;
case value2:
// Code to execute if expression matches value2
break;
// ... other cases
default:
// Code to execute if none of the above cases match
}
Example:
char grade = 'B';
switch (grade) {
case 'A':
printf("Excellent!\n");
break;
case 'B':
printf("Good job!\n");
break;
// ... other cases
default:
printf("Need improvement.\n");
}
Best Practices and Considerations
- Boolean Expressions: Use clear and concise boolean expressions in your conditions.
- Code Formatting: Indent your code within
if
,else
, andswitch
blocks for improved readability. - Error Handling: Consider
default
cases inswitch
statements to handle unexpected values. - Logical Operators: Use logical operators (
&&
,||
,!
) to combine multiple conditions.
FAQs: Decision Control Instructions in C
Q: Which decision control instruction is the most efficient?
A: The efficiency depends on the specific scenario. switch
statements can be faster than nested if-else
for multiple discrete cases, but the compiler optimization also plays a role.
Q: Can I nest if-else
statements within a switch
case?
A: Yes, you can create nested conditional logic within a switch
case if needed.
Q: Is there a limit to the number of case
statements in a switch
?
A: There’s no strict limit, but it’s good practice to keep the number of cases manageable for readability.
Q: What is the default
case in a switch
statement?
A: The default
case is optional and executes if none of the other case
values match the switch
expression.