Operator hierarchy, also known as operator precedence, is a fundamental concept in C programming. It dictates the order in which operators are evaluated within an expression, much like the order of operations in mathematics. Understanding this hierarchy is crucial for writing correct and predictable C code, especially when dealing with complex expressions. This comprehensive guide will break down C’s operator hierarchy, provide practical examples, and help you avoid common pitfalls.
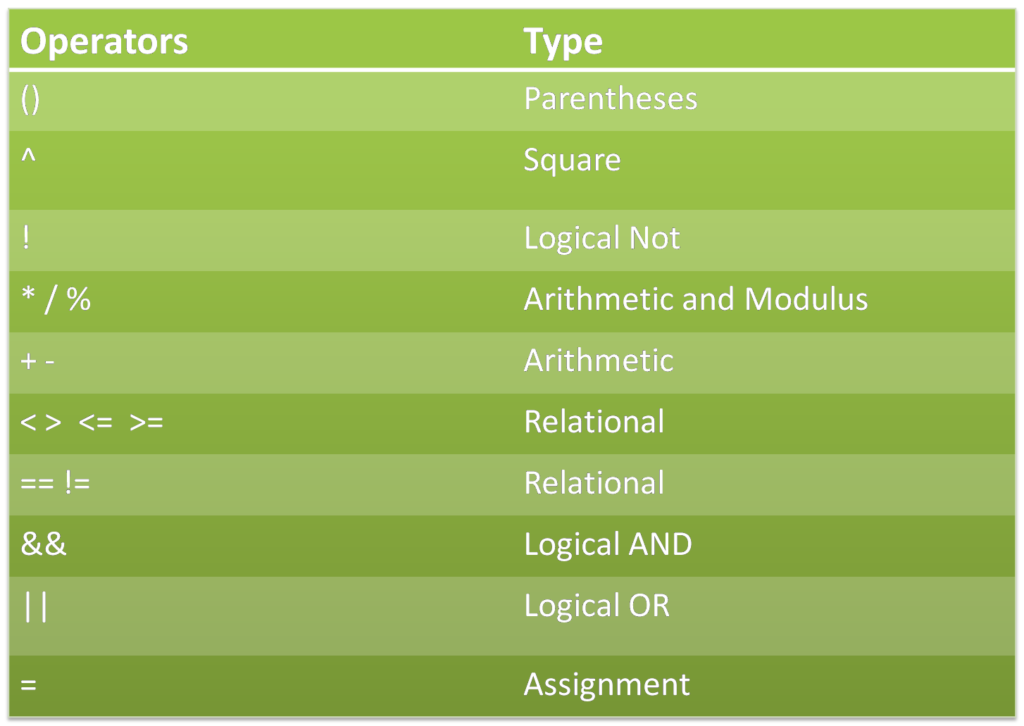
Understanding Operator Precedence
In C, operators are categorized into different levels of precedence. Operators with higher precedence are evaluated before those with lower precedence. This hierarchy ensures that expressions are evaluated consistently, regardless of the specific compiler used. Let’s delve into the various precedence levels, starting with the highest:
C Operator Precedence Table
Precedence Level | Operators | Associativity |
1 | () [] -> . ++ — | Left to right (postfix unary) |
2 | ! ~ + – ++ — (type) | Right to left (prefix unary) |
3 | * / % | Left to right |
4 | + – | Left to right |
5 | << >> | Left to right |
6 | && | Left to right |
7 | ||
8 | ?: | Right to left |
9 | #ERROR! | Right to left |
^= <<= >>= | ||
10 | , | Left to right |
Associativity
In addition to precedence, operators have associativity, which determines the order of evaluation for operators with the same precedence. Most operators associate from left to right, meaning they’re evaluated in the order they appear in the expression. However, some operators, like assignment and unary operators, associate from right to left.
Practical Examples
Let’s illustrate operator hierarchy with a few examples:
int x = 5 + 3 * 2; // x will be 11 (multiplication before addition)
int y = (5 + 3) * 2; // y will be 16 (parentheses override precedence)
int z = ++x; // x becomes 12, z becomes 12 (prefix increment)
int w = x++; // w becomes 12, x becomes 13 (postfix increment)
Common Pitfalls and How to Avoid Them
- Overlooking Precedence: A common mistake is assuming an incorrect order of evaluation. Always refer to the precedence table or use parentheses for clarity.
- Implicit Type Conversion: Be mindful of implicit type conversions, as they can affect the outcome of expressions.
- Side Effects: Operators with side effects (like ++ and –) can lead to unexpected behavior if not used carefully.
The Power of Parentheses
Parentheses are your best friend in C. They allow you to explicitly control the order of evaluation, making your code more readable and less prone to errors. Don’t hesitate to use parentheses whenever you’re unsure about operator precedence.
FAQ (Frequently Asked Questions):
Q: Can I modify the operator precedence in C?
A: No, the operator precedence in C is fixed and cannot be changed by the programmer.
Q: How does operator hierarchy impact code performance?
A: While operator hierarchy itself doesn’t directly affect performance, understanding it can help you write more efficient code by avoiding unnecessary operations.
Q: Are there any online tools for visualizing C operator precedence?
A: Yes, several websites and tools provide interactive visualizations of C’s operator precedence, making it easier to grasp.
Q: Is operator precedence the same in other programming languages?
A: Operator precedence can vary between programming languages. Always consult the specific language’s documentation.