Keywords are predefined reserved words in the C language that have a special meaning and cannot be used as variable names, function names, or any other identifiers in the program. These keywords are part of the syntax and grammar of the language and are used to define the structure of the program.
What Are Keywords?
- Keywords are reserved words in a programming language that hold predefined meanings and cannot be used for other purposes.
- They form the building blocks of the language’s syntax and convey specific instructions to the compiler or interpreter.
The Significance of Keywords in C
- Keywords provide a standardized vocabulary for expressing program logic.
- They ensure consistency and clarity in code comprehension, aiding both developers and compilers.
- C language offers a set of keywords, each serving a distinct purpose.
List of the keywords in C language
There are only 32 keywords available in C.
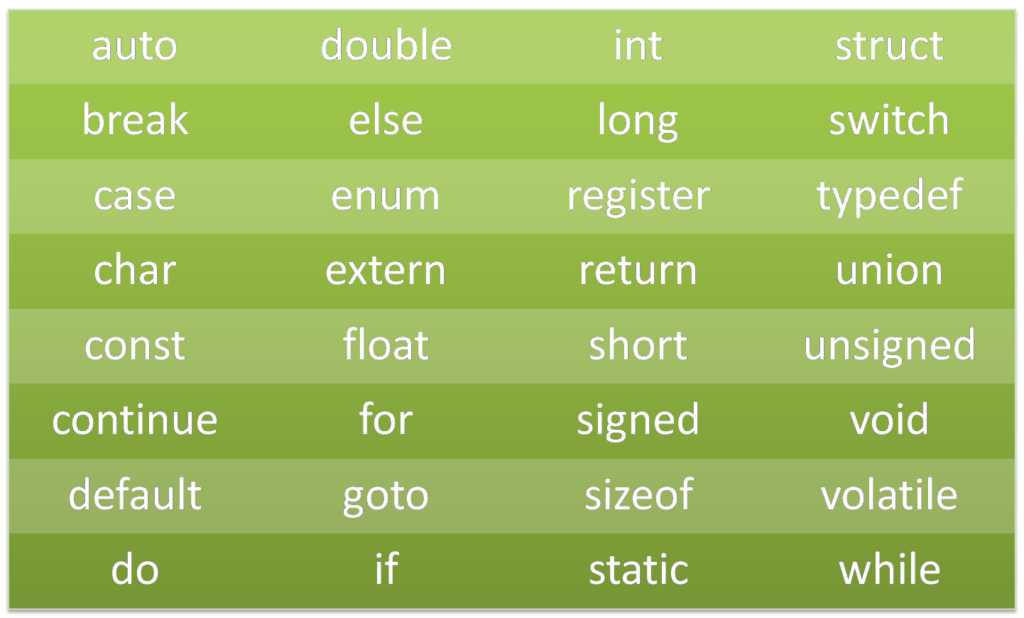
Here is a brief explanation of each keyword:
- auto – Specifies that a variable has automatic storage duration.
- break – Terminates the nearest enclosing loop or switch statement.
- case – Labels a statement within a switch statement.
- char – Specifies a character type.
- const – Specifies that a variable’s value cannot be changed.
- continue – Skips the rest of the loop body and continues with the next iteration.
- default – Specifies the default label in a switch statement.
- do – Starts a do-while loop.
- double – Specifies a double-precision floating-point type.
- else – Specifies an alternative to an if statement.
- enum – Specifies an enumeration type.
- extern – Specifies that a variable or function is defined in another file.
- float – Specifies a single-precision floating-point type.
- for – Starts a for loop.
- goto – Transfers control to another part of the program.
- if – Starts an if statement.
- int – Specifies an integer type.
- long – Specifies a long integer type.
- register – Specifies that a variable is stored in a register.
- return – Returns a value from a function.
- short – Specifies a short integer type.
- signed – Specifies a signed integer type.
- sizeof – Returns the size of a type or variable.
- static – Specifies that a variable or function has static storage duration.
- struct – Specifies a structure type.
- switch – Starts a switch statement.
- typedef – Specifies a type alias.
- union – Specifies a union type.
- unsigned – Specifies an unsigned integer type.
- void – Specifies a type with no value.
- volatile – Specifies that a variable may be changed by external factors.
- while – Starts a while loop.
Commonly Used Keywords in C:
- Data Types:
- int: Represents integer values.
- float: Denotes floating-point numbers.
- char: Defines single characters or small character arrays.
- double: Represents double-precision floating-point numbers.
- Control Flow:
- if/else: Facilitates conditional execution of code blocks.
- for/while: Enables loop iterations based on specific conditions.
- switch: Implements multi-branch decision making.
- break: Terminates a loop or switch case.
- Storage Class:
- auto: Specifies automatic storage duration.
- static: Preserves variable values across function calls.
- extern: Declares variables or functions in external files.
- Functions:
- return: Specifies the return value of a function.
- void: Indicates the absence of a return value.
- main: Marks the entry point of a C program.
Best Practices When Using Keywords
- Avoid using keywords as variable or function names.
- Use meaningful identifiers that reflect the purpose of your program elements.
- Familiarize yourself with the specific rules and restrictions associated with C language keywords.
Conclusion
Keywords in C language are indispensable tools for expressing program logic and ensuring code clarity and consistency. By understanding the significance and proper usage of keywords, developers can harness the full potential of the C language and create efficient and robust software.
As you embark on your coding journey, remember to leverage keywords wisely, following best practices and maintaining a strong grasp of their meanings and limitations. Embrace the power of keywords, and let your C programs shine with clarity and precision.