Dynamic memory allocation in C is a powerful technique in C programming that allows you to allocate and manage memory during the execution of your program. Unlike static memory allocation, where the size of variables is fixed at compile time, dynamic memory allocation enables you to request memory on demand, making your programs more adaptable and efficient. This guide will delve into the four essential functions used for dynamic memory allocation in C: malloc()
, calloc()
, realloc()
, and free()
, providing clear explanations, examples, and best practices.
Why Dynamic Memory Allocation Matters in C
Dynamic memory allocation empowers you to:
- Optimize Memory Usage: Allocate memory only when needed and release it when it’s no longer required, reducing waste.
- Handle Variable-Sized Data: Create data structures like linked lists, trees, and dynamically sized arrays, where the number of elements can vary.
- Improve Performance: Avoid the overhead of pre-allocating large chunks of memory that may not be fully utilized.
The 4 Core Functions for Dynamic Memory Allocation in C
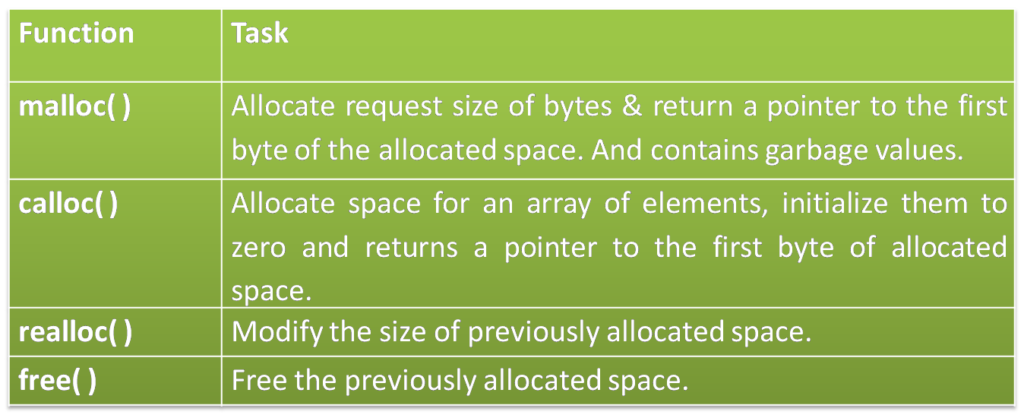
malloc()
(Memory Allocation):
- Reserves a specified block of memory in the heap.
- Returns a
void
pointer to the first byte of the allocated memory. - Memory is uninitialized (contains garbage values).
- Syntax:
ptr = (cast_type*) malloc(byte_size);
calloc()
(Contiguous Allocation):
- Similar to
malloc()
, but initializes the allocated memory to zero. - Often used for allocating arrays.
- Syntax:
ptr = (cast_type*) calloc(n, element_size); // Allocates memory for 'n' elements of 'element_size'
realloc()
(Re-Allocation):
- Changes the size of a previously allocated memory block.
- Can potentially move the block to a new location if resizing is not possible in place.
- Syntax:
ptr = realloc(ptr, new_size);
free()
(Deallocate Memory):
- Releases a previously allocated block of memory back to the heap, making it available for reuse.
- Syntax:
free(ptr);
Best Practices and Error Handling
- Always Check Return Values:
malloc()
,calloc()
, andrealloc()
returnNULL
if memory allocation fails. Always check forNULL
before using the returned pointer. - Free Memory When No Longer Needed: Prevent memory leaks by calling
free()
when you are finished using the allocated memory. - Use the Right Size: Allocate the appropriate amount of memory. Using too little can lead to buffer overflows, while using too much wastes resources.
- Handle Reallocation Carefully: Be aware that
realloc()
might return a different memory address than the original pointer. Update your pointers accordingly.
FAQs: Dynamic Memory Allocation in C
Q: Why is dynamic memory allocation important in C?
A: Dynamic memory allocation offers flexibility, allowing you to allocate memory as needed during program execution, handling variable-sized data, and optimizing memory usage.
Q: How do I decide which function (malloc()
, calloc()
, or realloc()
) to use?
A: Use malloc()
when you need a block of uninitialized memory. Use calloc()
for allocating and initializing arrays to zero. Use realloc()
to change the size of an existing block.
Q: What happens if I forget to free memory in C?
A: This can lead to a memory leak, where your program gradually consumes more and more memory until it runs out, potentially causing crashes or performance issues.