Application of Queue is a fundamental concept in computer science and real life. A queue is a linear data structure based on the First-In, First-Out (FIFO) principle—just like people lining up at a store. Beyond simple lines, queues are vital for managing processes, tasks, and resources efficiently in modern systems.
How Queues Work
A queue has two main ends:
- Front (Head): Where elements are removed (dequeued).
- Rear (Tail): Where elements are added (enqueued).
It maintains FIFO behavior, ensuring the first inserted element is the first to be processed.
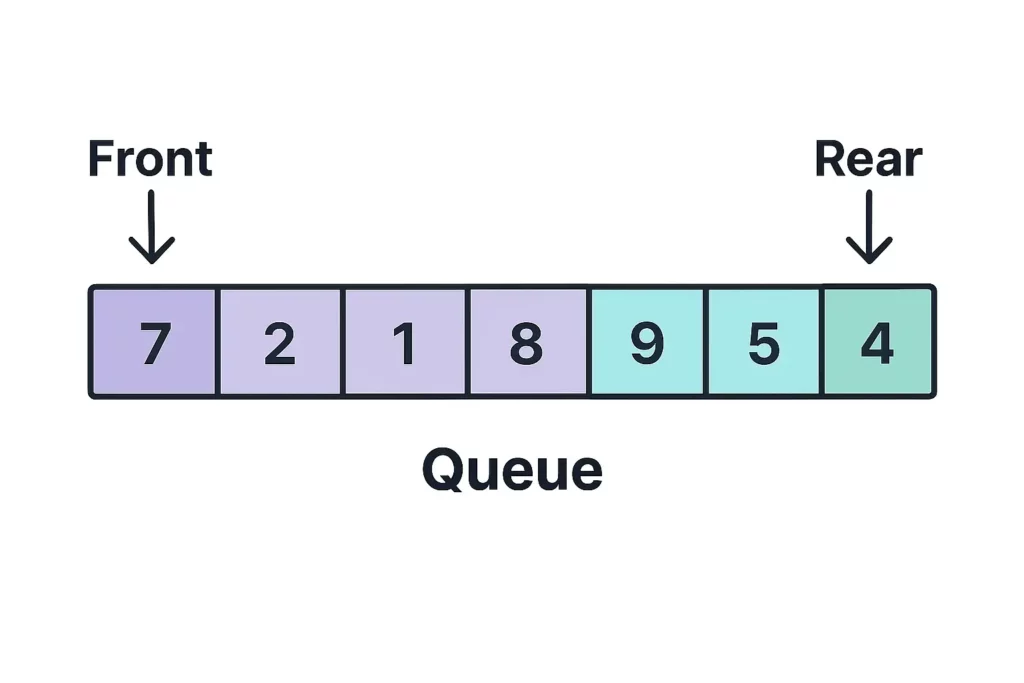
Types of Queues
Understanding different queue types helps you apply the right one for your scenario.
1. Simple Queue
The basic structure where insertion happens at the rear and deletion at the front. Used in scheduling and buffers.
2. Circular Queue
Connects the end back to the start, saving space in a fixed-size array. Ideal for memory-efficient applications.
3. Priority Queue
Each element has a priority. Higher-priority elements are processed first. Used in OS scheduling, Dijkstra’s algorithm, and emergency response systems.
4. Double-Ended Queue (Deque)
Insertion and deletion are allowed from both ends. Useful in palindrome checks, undo operations, and task scheduling.
Application of Queue in Daily Life
1. Customer Service Systems
In banks or customer service centers, customers are queued and served in order. Queues ensure fairness and systematic service delivery.
2. Traffic Light Systems
Vehicles at intersections follow a queue mechanism controlled by traffic signals, ensuring ordered and safe movement.
3. Call Centers
Incoming calls are placed in a queue, waiting to be answered by the next available agent. This maintains orderly call management.
Application of Queue in Computer Science
1. CPU and Process Scheduling
Operating systems use queues to manage process scheduling. Each process waits in a queue for its turn based on priority or arrival time.
2. Printer Spooling
Print jobs are stored in a queue. The printer fetches one job at a time, maintaining the correct print order.
3. I/O Buffers and Data Streaming
Audio and video buffers in streaming platforms use queues to manage continuous data flow, ensuring smooth playback.
4. Breadth-First Search (BFS) in Graphs
BFS uses queues to track which nodes to visit next. It’s used in pathfinding algorithms, social network analysis, and network broadcasting.
5. Task Queues in Asynchronous Programming
Languages like JavaScript use queues to handle asynchronous tasks, ensuring order in event handling and background execution.
Modern Applications of Queues in Technology
1. Cloud-Based Microservices
Message queues (e.g., RabbitMQ, AWS SQS) allow distributed services to communicate asynchronously, improving scalability and reliability.
2. Load Balancing and Job Queuing
Web servers use queues to handle incoming requests. Load balancers distribute them to avoid overloading any single server.
3. Real-Time Systems and Simulations
In robotics and simulations, event queues handle real-time data, controlling system responses and actions.
4. Multimedia Systems
Queues manage frames in video rendering pipelines, ensuring synchronization and smooth visual output.
Common Operations on Queues
Understanding these basic operations helps in implementing and manipulating queues effectively:
- Enqueue: Add element at the rear.
- Dequeue: Remove element from the front.
- Peek: View front element without removing it.
- isEmpty: Check if the queue is empty.
- isFull: For fixed-size queues, check if the queue is full.
Programming Language Implementations
Language | Queue Library/Module | Notes |
---|---|---|
Python | collections.deque | Thread-safe and efficient for most cases. |
Java | java.util.Queue | Interface with implementations like LinkedList and PriorityQueue. |
C++ | std::queue , std::deque | Supports custom containers and types. |
JavaScript | Custom Array Logic | Often implemented via shift and push. |
Recommended Books for Queue and Data Structures
- Data Structures and Algorithms Made Easy by Narasimha Karumanchi
- Data Structures and Algorithms in Python by Goodrich, Tamassia, Goldwasser
These books offer in-depth knowledge and examples for queue implementation and applications in real-world scenarios.
Frequently Asked Questions: Application of Queue
Q1. What is the main application of queue in computing?
Queues are widely used in CPU scheduling, printer spooling, and asynchronous task management, ensuring orderly task execution.
Q2. Why are circular queues preferred over simple queues?
Circular queues reuse memory efficiently, avoiding wastage when the queue has space at the front but not at the rear.
Q3. What is the use of priority queues?
Priority queues are used in emergency response systems, OS scheduling, and algorithms like Dijkstra’s, where urgency matters more than arrival order.
Q4. Can queues be implemented using linked lists?
Yes, queues can be implemented using arrays or linked lists. Linked lists offer dynamic sizing, while arrays are faster but fixed in size.
Q5. How are queues used in real-time systems?
Real-time systems like robotics, media players, and simulations use queues to process events and maintain consistent performance.
Conclusion: Mastering the Application of Queue
Understanding the Application of Queue is vital for both theoretical learning and practical development. From managing traffic to processing jobs in an operating system, queues are everywhere. Whether in customer service, cloud computing, or data streaming, queues provide efficient, fair, and structured handling of tasks.
With the rise of cloud-native architectures, AI workloads, and real-time systems, queues continue to play a central role in designing scalable and high-performance applications. Mastering queues equips you with the tools to build smarter, faster, and more organized solutions.