Best of luck with your upcoming interview, data structure is one of the important subjects where the interviewer asks questions.
This article contain almost every interview question asked in data structure.
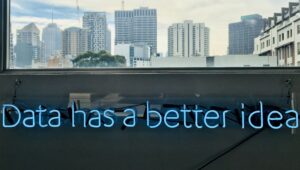
Data structure interview questions Set1(1-10):
1. What do you mean by Data Structure?
Ans. The logical or mathematical model of a particular organization of data is called a data structure.
2. What is indirection?
Ans. If you have a pointer to a variable or any other object in memory, you have an indirect reference to its value. and * is used to apply indirection.
3. What is a null pointer?
Ans. There are times when it’s necessary to have a pointer that doesn’t point to anything. The macro NULL, defined in , has a value that’s guaranteed to be different from any valid pointer. NULL is a literal zero.
4. When is a void pointer used?
Ans. A void pointer is used for working with raw memory or for passing a pointer to an unspecified type.
5. What is the heap?
Ans. The heap is where malloc(), calloc(), and realloc() get memory.
6. What is the difference between “calloc(…)” and malloc(…)?
Ans. calloc(…) allocates a block of memory for an array of elements of a certain size. malloc(…) takes in only a single argument which is the memory required in bytes.
7. How a data structure is Represented?
Ans. Any data structure can be represented using Sequential representation & Linked representation.
8. How are pointer variables initialised?
Ans. A Pointer can be initialised either by Static memory allocation or Dynamic memory allocation.
9. What is Row Major Ordering in array?
Ans. Row major ordering assigns successive elements, moving across the rows and then down the columns, to successive memory locations.
10. What is Column Major Ordering ?
Ans. If the Element of the array is being stored in Column Wise fashion then it is called column-major ordering in the array.
Data structure interview questions Set2(11-20):
11. What is STACK?
Ans. A stack is a linear structure in which items may be added or removed only at one end called Top of the stack.
12. Tell the application of stack?
Ans. Stack is internally used by the compiler when we implement (or execute) any recursive function and to evaluate a mathematical expression and check the parentheses in an expression.
13. What is QUEUE?
Ans. A queue is a linear list of elements in which deletion can take place only at one end, called the FRONT, and insertions can take place only at the other end, called the REAR. It is also called First-in First-out (FIFO) lists.
14. Why Stack Segment is used?
Ans. The variable that is defined inside the block/local scope without using a static storage class is stored in the stack segment.
15. Does heap grow up or down?
Ans. Heap always grows down.
16. What is a pointer value and address?
Ans. A pointer value is a data object that refers to a memory location. Each memory location is numbered in the memory. The number attached to a memory location is called the address of the location.
17. Give difference between sturcture and union?
Ans. All the members of the structure can be accessed at once, whereas in a union only one member can be used at a time.
18. How referencing and dereferencing is done with pointers?
Ans. Providing reference we write pointer name followed by assignment operator then we write or provide an address. Pointer_Name = Address of memory(or location). For example Int *p; P = &i; // This is called referencing. Pointer is dereferenced using asterisk or dereference operator for example *p;
19. What do you mean by call by reference?
Ans. When the address of the actual argument is passed to the formal argument at the time of function call, called call by reference. For example swap(&a, &b).
20. By which functions memory management is done?
Ans. Memory management in c is done using calloc( ), malloc( ), realloc( ) and free( ) functions. These functions can allocate, reallocate, deallocate, and free memory during runtime. Allocation, deallocation of memory during runtime is known as dynamic memory management.
Data structure interview questions Set3(21-30):
21. When memory is allocated in static memory allocation and dynamic memory allocation?
Ans. In static memory allocation, memory is assigned during compile time and in dynamic memory allocation, memory is assined during run time.
22. What do you mean by Code Segment:?
Ans. Whole program code is stored in code segment.
23. What happened in INSERTION SORT?
Ans. Suppose an array A with n elements A[0], A[1], A[2], . . . . . , A[n-1] is in memory. The insertion sort algorithm scans A from A[1] to A[n-1], Inserting each element into its proper position.
24. What happened in SELECTION SORT:?
Ans. In selection At first shortest element is selected and then inserted to the selected location 0. Then next shortest element is selected and inserted to selected location 1. And so on…….
25. What do you mean by Linked List?
Ans. The link list is a collection of elements and each element is called a node. Where each node divided into two parts. The first part contains information of the element and the second part called the link field or next pointer field, contains the address of the next node in the list.
26. What do you mean by TREE in data structure?
Ans. The tree structure is mainly used to represent data containing a hierarchal relationship between elements.
27. Give the definition of Root?
Ans. Root is the first node in the hierarchical arrangement of the data items.
28. What do you mean by internal node?
Ans. An internal node is any node of a tree that has child nodes.
29. What do you mean by external node?
Ans. An external node is any node that does not have child nodes.
30. What is height of a node?
Ans. The height of a node is the length of the longest downward path to a leaf from that node.
Data structure interview questions Set4(31-40):
31. What is depth?
Ans. The depth of a node is the length of the path to its root (i.e., its root path).
32. What do you mean by subtree?
Ans. A subtree of a tree T is a tree consisting of a node in T and all of its descendants in T.
33. List out the areas in which data structures are applied extensively?
Ans. Compiler Design, Operating System, DBMS, Numerical Analysis, Graphics, AI, Simulation
34. What are the major data structures used in the following areas: RDBMS, Network data model, and Hierarchical data model?
Ans. RDBMS(Array of Structure), Network Data Model(Graph), Hierarchical Data Model(Tree).
35. If you are using C language to implement the heterogeneous linked list, what pointer type will you use?
Ans. Void Pointer
36. Minimum number of queues needed to implement the priority queue?
Ans. Two. One queue is used for the actual storing of data and another for storing priorities.
37. What is the data structures used to perform recursion?
Ans. Stack. Because of its LIFO (Last In First Out) property, it remembers its ‘caller’ so knows whom to return when the function has to return. Recursion makes use of the system stack for storing the return addresses of the function calls.
38. What are the notations used in the Evaluation of Arithmetic Expressions using prefix and postfix forms?
Ans. Polish and Reverse Polish notations.
39. Sorting is not possible by using which of the following methods?
Ans. (Insertion, Selection, Exchange, Deletion) Sorting is not possible in Deletion. Using insertion we can perform insertion sort, using selection we can perform selection sort, using exchange we can perform the bubble sort (and other similar sorting methods). But no sorting method can be done just using deletion.
40. What are the methods available in storing sequential files ?
Ans. Straight Merging, Natural Merging, Polyphase Sort, Distribution of Initial Sort.
Data structure interview questions Set5(41-48):
41. List out few of the Application of tree data-structure?
Ans. Manipulation of Arithmetic expression, Symbol Table, Syntax analysis.
42. List out few of the applications that make use of Multilinked Structures?
Ans. Sparse Matrix, Index Generation.
43. In tree construction which is the suitable efficient data structure?
Ans. (Array, Linked list, Stack, Queue) A linked list is a suitable efficient data structure.
44. What is the type of the algorithm used in solving the 8 Queens problem?
Ans. Backtracking
45. In an AVL tree, at what condition the balancing is to be done?
Ans. If the ‘pivotal value’ (or the ‘Height factor’) is greater than 1 or less than -1.
46. What is the bucket size, when the overlapping and collision occur at same time?
Ans. One. If there is only one entry possible in the bucket, when the collision occurs, there is no way to accommodate the colliding value. This results in the overlapping of values.
47. In RDBMS, what is the efficient data structure used in the internal storage representation?
Ans. B+ tree. Because in the B+ tree, all the data is stored only in leaf nodes, that makes searching easier. This corresponds to the records that shall be stored in leaf nodes.
48. What is a spanning Tree?
Ans. A spanning tree is a tree associated with a network. All the nodes of the graph appear on the tree once. A minimum spanning tree is a spanning tree organized so that the total edge weight between nodes is minimized.
We hope you liked the above data structure interview questions, if you find any mistakes then you can simply comment below.